お久しぶりです。Sanagiです。
今回はSpring BootでController↔︎View間で値の受け渡し方法を書いていきます。
Spring Boot初心者の方はぜひ参考にしていただければ幸いです!
前提
OS:Mac
Javaバージョン:21
Spring Bootバージョン:3.2.0
thymeleafバージョン:3.1.2
Controller→HTMLへ渡す方法
Hello.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Hello</title>
</head>
<body>
<p><span th:text="${name}"></span>さん。こんにちは。</p>
</body>
</html>
Sample.Java
package com.example.demo;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class Sample {
@RequestMapping("/hello")
public String exec(Model model) {
model.addAttribute("name", "田中");
return "Hello";
}
}
実行結果
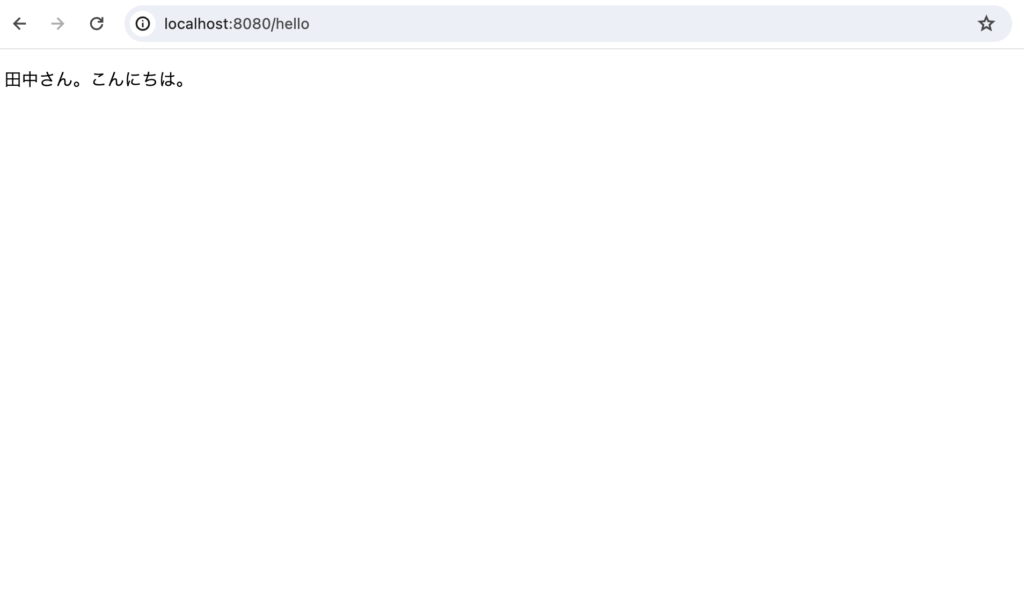
Controller→JavaScriptへ渡す方法
Hello2.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Hello2</title>
</head>
<body>
<p><span id="test">佐藤さん</span></p>
</body>
<script th:inline="javascript">
var val = document.getElementById("test");
val.innerText = /*[[${name}]]*/''
</script>
</html>
Sample2.Java
package com.example.demo;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class Sample2 {
@RequestMapping("/hello2")
public String exec(Model model) {
model.addAttribute("name", "斉藤さんに変えました。");
return "Hello2";
}
}
実行結果
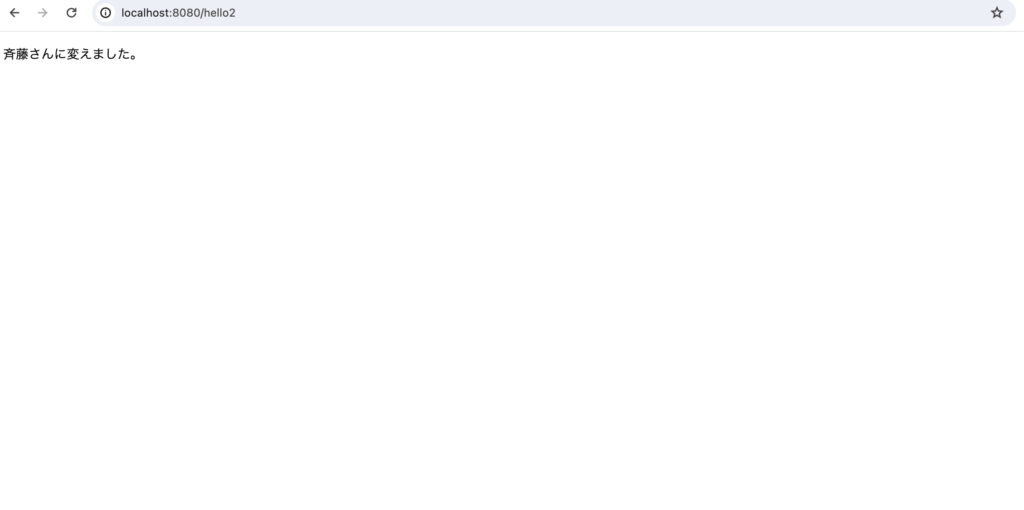
HTML→Controllerへ渡す方法(GET&POST)
Hello3.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Hello3</title>
</head>
<body>
<!-- GET -->
<form action="/hello4" method="get">
<input name="nameget"> <button type="submit">送信(GET)</button>
</form>
<!-- POST -->
<form action="/hello4" method="post">
<input name="namepost"> <button type="submit">送信(POST)</button>
</form>
</body>
</html>
Hello4.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Hello4</title>
</head>
<body>
<p><span th:text="${name}"></span>さん。こんにちは。</p>
</body>
</html>
Sample3.Java
package com.example.demo;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
@Controller
public class Sample3 {
@RequestMapping("/hello3")
public String exec(Model model) {
return "Hello3";
}
@GetMapping("/hello4")
public String exec2(Model model, @RequestParam("nameget")String nameget) {
model.addAttribute("name", nameget);
return "Hello4";
}
@PostMapping("/hello4")
public String exec3(Model model, @RequestParam("namepost")String namepost) {
model.addAttribute("name", namepost);
return "Hello4";
}
}
実行結果
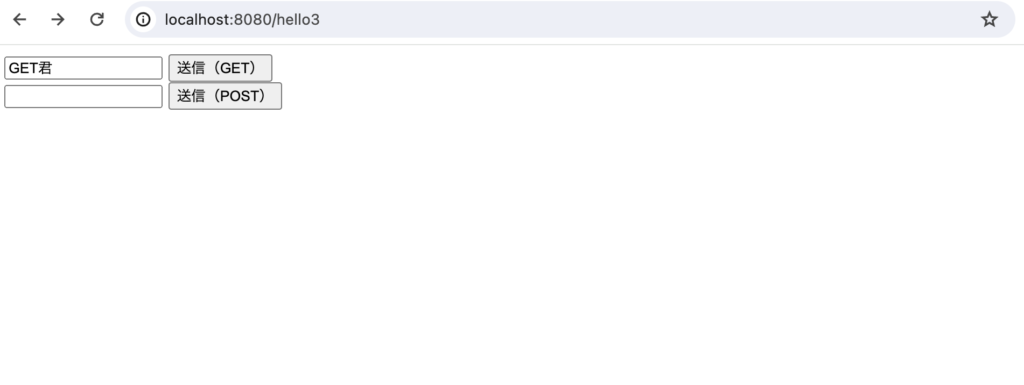
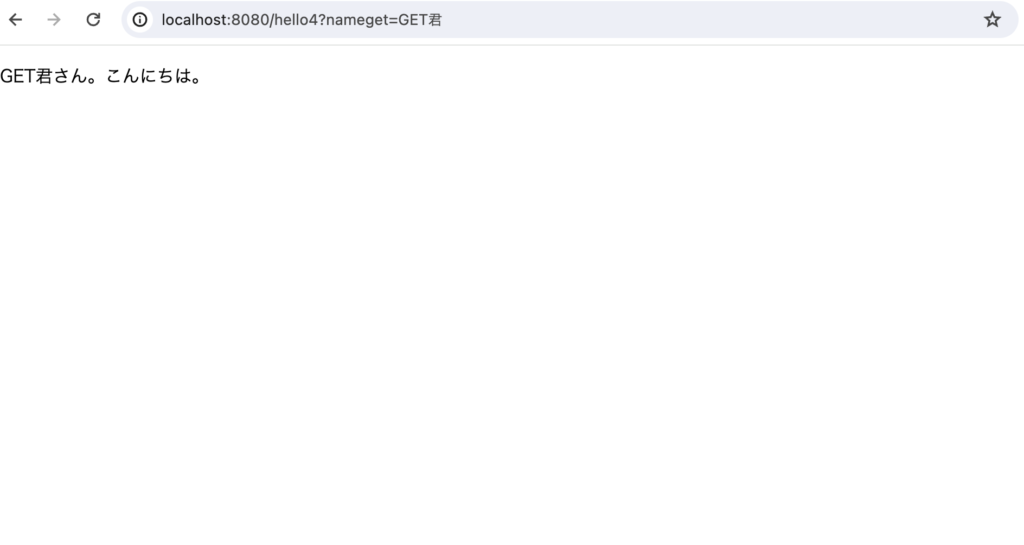
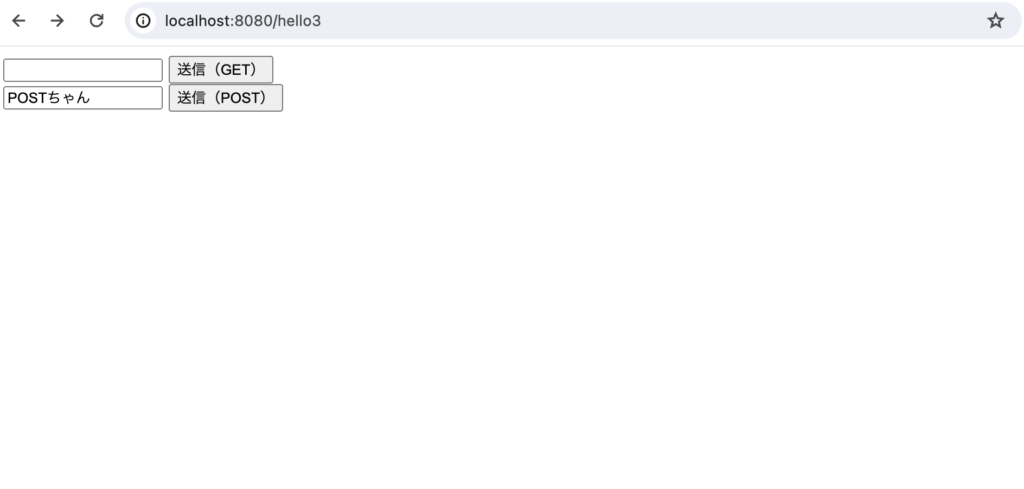
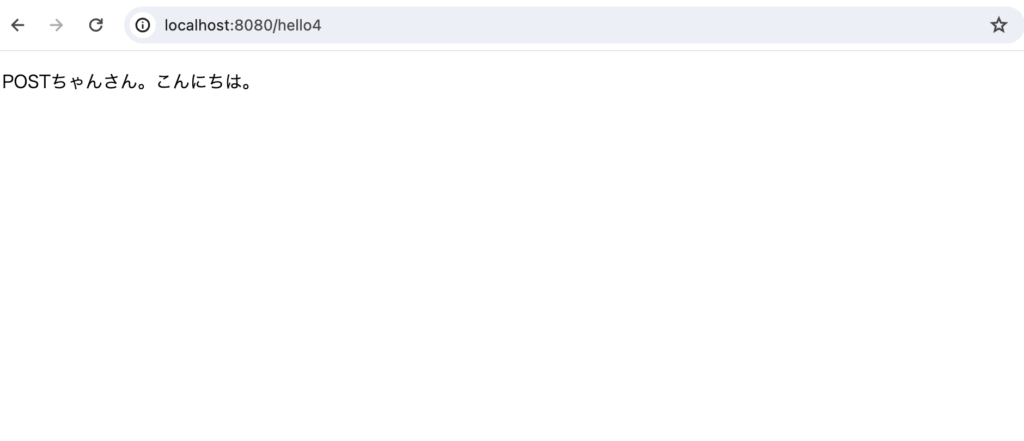
以上。